In C language, the strcoll()
function compares two strings based on the current locale settings. Unlike strcmp()
, strcoll()
considers localized character sorting rules, whereas strcmp()
compares based on ASCII values.
Reference
strcoll Function Header
The header file for the strcoll
function is <string.h>
. Before using the strcoll
function, make sure to include this header file in your C code:
#include <string.h>
strcoll Function Prototype
int strcoll(const char *s1, const char *s2);
The strcoll
function compares the string pointed to by s1
with the string pointed to by s2
, interpreting both strings according to the LC_COLLATE
category of the current locale.
The strcoll()
function depends on the current locale settings. It may yield different results in different locales. If the locale is not set correctly or is unsupported, strcoll()
might default to the C language environment for comparison.
On Linux systems, you can check supported locales using the locale -a
command:
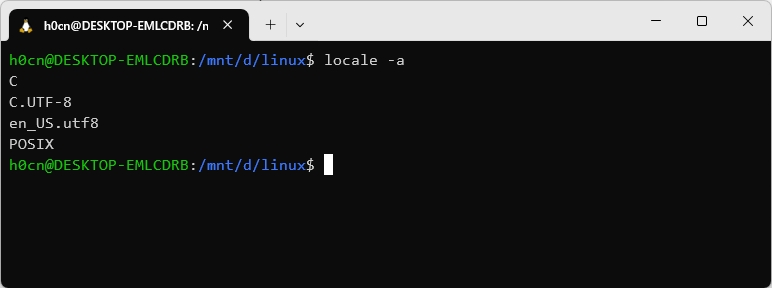
Function Parameters
s1
: Pointer to the first string;s2
: Pointer to the second string;
Return Value
The strcoll
function returns an integer greater than, equal to, or less than 0
;
If the two strings are identical, it returns 0
;
strcoll Example Code
If the locale en_US.utf8
is unsupported on your system, the comparison results of strcmp()
and strcoll()
might be the same;
#include <stdio.h>
#include <string.h>
#include <locale.h>
int main() {
// Define strings with regular and accented characters
char str1[] = "café";
char str2[] = "cafe";
// Compare using strcmp
int strcmp_result = strcmp(str1, str2);
// Set locale to en_US.utf8 (US English locale)
setlocale(LC_COLLATE, "en_US.utf8");
// Compare using strcoll
int strcoll_result_us = strcoll(str1, str2);
printf("strcmp result: %d\n", strcmp_result);
printf("strcoll result (en_US.utf8 locale): %d\n", strcoll_result_us);
return 0;
}
Rerun Output
strcmp result: 94 strcoll result (en_US.utf8 locale): 1